Time Complexity
Author:
@wkw
Overview
Time Complexity is one of the important measurements when it comes to writing an efficient solution. It estimates how much time your solution needs based on some input. If your solution is too slow, even it passes some test cases, it will still consider it as a wrong answer.
The time complexity is denoted by Big O notation. Normally, means the input size. For example, if the input is a string , then would be the length of .
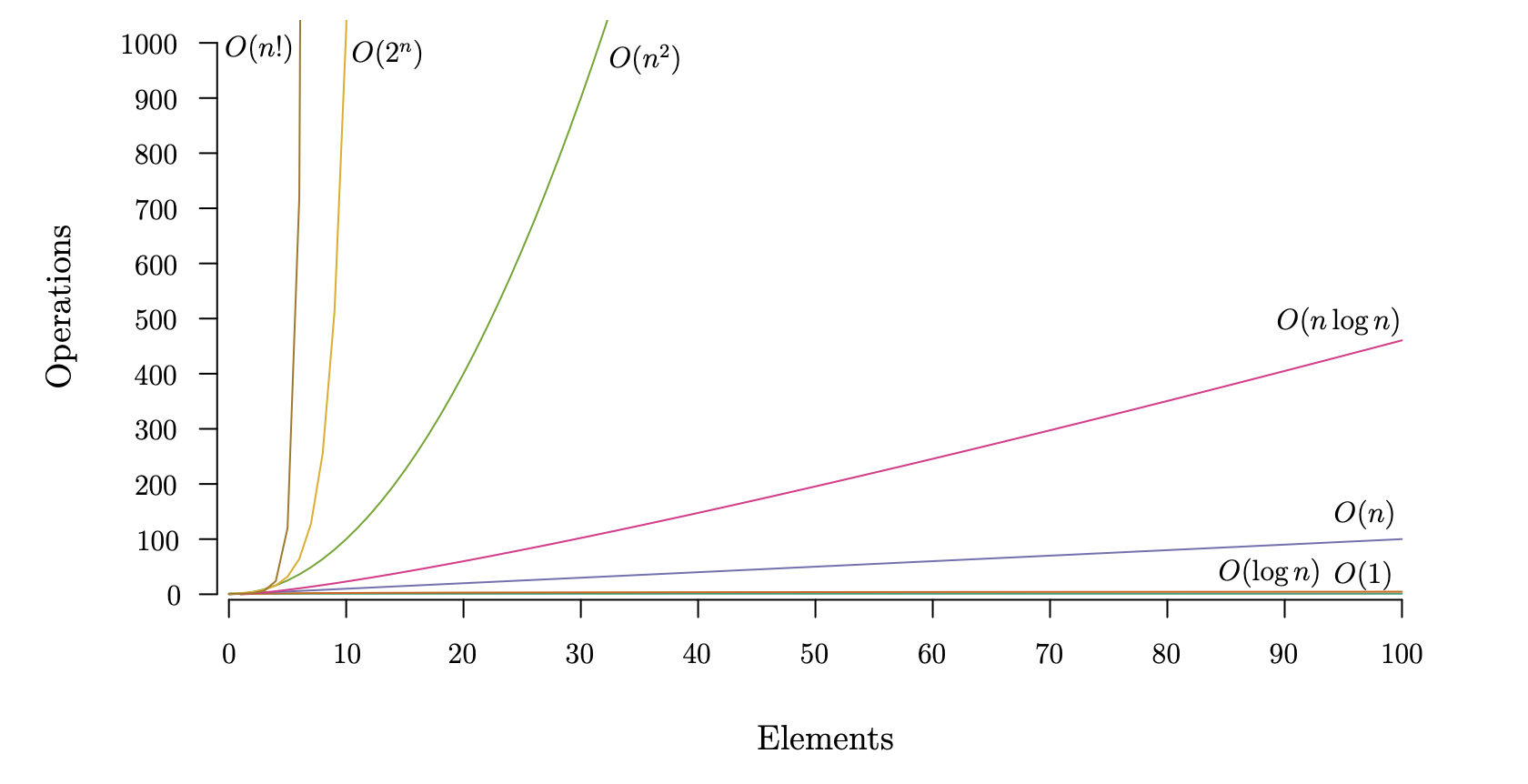
Estimating Time Complexity
Input size | Time Complexity |
---|---|
, | |
, |
Example 1:
In the following case, the time complexity depends on . Therefore, it is .
for (int i = 0; i < n; i++) {
// do something
}
Example 2:
In the following case, the time complexity depends on and . Therefore, it is .
for (int i = 0; i < n; i++) {
for (int j = 0; j < m; j++) {
// do something
}
}
Example 3:
In the following case, the time complexity is . You can see as .
As sqrt() returns double, it would be safe to use to check the condition instead of using .
for (int i = 2; i * i <= n; i++) {
// do something
}